Part 1: Database
On this part we are going to create a database named employeedb.
1. Run
as Administrator.
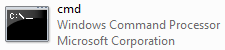
2. Type cd %JAVA_HOME%\db\bin & ij.bat to open the Apache Derby CLI.
3. Execute the below commands...
![]() |
http://pastie.org/4926035 |
4. Type exit;, to quit.
Part 2: Servlet
On this part we are going to create a servlet named Employee.
1. Run
.
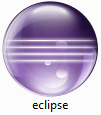
2. On the eclipse Juno IDE press Alt + Shift + N then choose

3. The 'New Dynamic Web Project' dialog will show, name the project as EmployeeInfoWeb, then hit


3. Again accept the defaults, hit

4. On the 'Project Explorer' right click the

5. The 'Create Servlet' dialog will show, name the servlet as Employee, just hit
to end the wizard.






package com.empinfo;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class Employee
*/
@WebServlet("/Employee")
public class Employee extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* Default constructor.
*/
public Employee() {
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/plain; charset=ISO-8859-1");
PrintWriter out = response.getWriter();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try{
Class.forName("org.apache.derby.jdbc.ClientDriver").newInstance();
conn = DriverManager.getConnection("jdbc:derby://localhost:1527/employeedb;");
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM employee_record WHERE id=" + request.getParameter("id").toString());
rs.next();
String fname = rs.getString("fname");
String lname = rs.getString("lname");
out.println(lname + ", " + fname);
out.close();
} catch (Exception e) {
out.println(e.toString());
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
}
8. Now, On the toolbar click
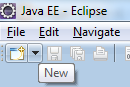
9. The 'New' dialog will show, choose 'Server', then hit


11. Add the current project to the server, then hit

12. Start Tomcat!
Part 3: Mobile App
1. Open
.
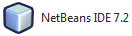
2. Click
to create a new project.

3. On the New Project dialog choose Java ME from the Categories then choose Mobile Application as the project type, then hit
.

4. Type EmployeeInfoMobile as project name, then hit
.

5. Enter the code below...
package hello;
import java.io.DataInputStream;
import javax.microedition.io.Connector;
import javax.microedition.io.HttpConnection;
import javax.microedition.lcdui.*;
import javax.microedition.midlet.MIDlet;
/**
* @author Jeremiah G. Gatong
*/
public class Midlet extends MIDlet implements CommandListener {
private Form f;
private Command find = new Command("Find", Command.OK, 1);
private TextField id;
private StringItem label;
public void main() {
f = new Form("Employee Information");
id = new TextField("Enter ID", "", 25, TextField.ANY);
label = new StringItem("", "");
f.append(id);
f.append(label);
f.addCommand(find);
f.setCommandListener(this);
}
public void startApp() {
main();
Display.getDisplay(this).setCurrent(f);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command c, Displayable d) {
if (c.equals(find)) {
StringBuffer sb;
try {
String url = "http://localhost:8080/EmployeeInfoWeb/Employee?id=" + id.getString();
HttpConnection cc = (HttpConnection) Connector.open(url);
cc.setRequestMethod(HttpConnection.GET);
cc.setRequestProperty("Content-Type", "text/plain");
DataInputStream in = (DataInputStream) cc.openDataInputStream();
int ch;
sb = new StringBuffer();
while ((ch = in.read()) != -1) {
sb.append((char) ch);
}
String str = sb.toString();
label.setText(str);
} catch (Exception e) {
System.err.println("Error :" + e);
}
}
}
}
Output