using Microsoft.Win32;
namespace USB
{
class Program
{
enum Permission { Allow = 3, Deny = 4 };
static void Main(string[] args)
{
RegistryKey key;
key = Registry.LocalMachine.OpenSubKey(@"SYSTEM\CurrentControlSet\services\USBSTOR", true);
key.SetValue("Start", (int)Permission.Deny);
}
}
}
*** Using the Microsoft.Win32 namespace you will be able to retrieve and modify the HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\services\USBSTOR registry key, Setting the value data of Start to 4 will disable the USB drives of the PC.
0-day
Friday, January 4, 2013
Disable USB drives using C#
The C# code below is capable of enabling/disabling the USB drives of computers running in Windows XP, Windows Vista or Windows 7.
Sunday, December 16, 2012
NET VIEW and C#
Part I: TERMS
What is NET VIEW command?
It's a Windows shell command that display a list of computers visible on the network. To use the command just press the winkey, type cmd.exe, hit enter, and on the command prompt just type NET VIEW, see below for the result.
What is the Standard Output?
Standard output is the stream where a program writes its output data (e.g. screen, file, etc...).
What is NET VIEW command?
It's a Windows shell command that display a list of computers visible on the network. To use the command just press the winkey, type cmd.exe, hit enter, and on the command prompt just type NET VIEW, see below for the result.
What is the Standard Output?
Standard output is the stream where a program writes its output data (e.g. screen, file, etc...).
Part II: THE APPLICATION
Objective
Build a C#.NET Console Application that is able to read the standard output stream of the NET VIEW command to show a list of connected computers in a network.
Project
1. Click on
.
2. Choose
from the start menu.

2. Choose

3. On Visual Studio choose File, New, and then Project.
4. The New Project dialog will appear, name the project as 'NetView', then hit
.
5. Navigate to the Solution Explorer, Right click the NetView project, select Add, and then choose Class.
6. The Add New Item dialog will show, name it as PC, then click 
7. Enter the code below for the PC.cs...
8. Enter the code below for the Program.cs...
Output
4. The New Project dialog will appear, name the project as 'NetView', then hit

5. Navigate to the Solution Explorer, Right click the NetView project, select Add, and then choose Class.

7. Enter the code below for the PC.cs...
namespace NetView
{
public class PC
{
public string ServerName { get; set; }
}
}
8. Enter the code below for the Program.cs...
using System;
using System.Collections.Generic;
using System.Text;
namespace NetView
{
class Program
{
static void Main(string[] args)
{
try
{
var pcs = DisplayComputers();
if (pcs != null)
{
foreach (var pc in pcs)
Console.WriteLine(pc.ServerName);
}
else
Console.WriteLine("Err Msg: There Are No Entries in the List");
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
}
Console.ReadKey();
}
/// <summary>
/// This method will display the list of computers in the current workgroup.
/// </summary>
/// <returns>List of computers</returns>
public static List<PC> DisplayComputers()
{
// Create a child process
System.Diagnostics.Process process = new System.Diagnostics.Process();
// Starts a new instance of the Windows command interpreter
process.StartInfo.FileName = "cmd.exe";
// Carries out the NET VIEW command and then terminates
process.StartInfo.Arguments = "/c net view";
process.StartInfo.CreateNoWindow = false;
// Redirect the output stream of the child process.
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
// Start!
process.Start();
List<PC> pcs = new List<PC>();
StringBuilder pc;
// Read the output stream
while (!process.StandardOutput.EndOfStream)
{
// This is the current stream data
string line = process.StandardOutput.ReadLine();
// ref: http://support.microsoft.com/kb/103013
if (line == "There are no entries in the list.")
return null;
// Find/Parse the pc name
if (line != string.Empty)
{
if (line[0] == '\\' && line[1] == '\\')
{
pc = new StringBuilder();
for (int y = 2; y < line.Length; y++)
{
if (line[y] == ' ')
break;
else
pc.Append(line[y]);
}
// Add the current PC to the PC list
pcs.Add(new PC { ServerName = pc.ToString() });
}
}
}
// Wait indefinitely for the associated process to exit
process.WaitForExit();
// Frees all the resources
process.Close();
return pcs;
}
}
}
Output
Sunday, October 7, 2012
J2ME Mobile Application Raw Http Call to a Servlet to query Derby Network Server
Part 1: Database
On this part we are going to create a database named employeedb.
1. Run
as Administrator.
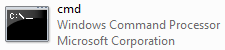
2. Type cd %JAVA_HOME%\db\bin & ij.bat to open the Apache Derby CLI.
3. Execute the below commands...
![]() |
http://pastie.org/4926035 |
4. Type exit;, to quit.
Part 2: Servlet
On this part we are going to create a servlet named Employee.
1. Run
.
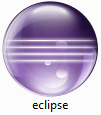
2. On the eclipse Juno IDE press Alt + Shift + N then choose

3. The 'New Dynamic Web Project' dialog will show, name the project as EmployeeInfoWeb, then hit


3. Again accept the defaults, hit

4. On the 'Project Explorer' right click the

5. The 'Create Servlet' dialog will show, name the servlet as Employee, just hit
to end the wizard.






package com.empinfo;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class Employee
*/
@WebServlet("/Employee")
public class Employee extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* Default constructor.
*/
public Employee() {
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/plain; charset=ISO-8859-1");
PrintWriter out = response.getWriter();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try{
Class.forName("org.apache.derby.jdbc.ClientDriver").newInstance();
conn = DriverManager.getConnection("jdbc:derby://localhost:1527/employeedb;");
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM employee_record WHERE id=" + request.getParameter("id").toString());
rs.next();
String fname = rs.getString("fname");
String lname = rs.getString("lname");
out.println(lname + ", " + fname);
out.close();
} catch (Exception e) {
out.println(e.toString());
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
}
8. Now, On the toolbar click
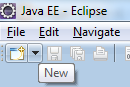
9. The 'New' dialog will show, choose 'Server', then hit


11. Add the current project to the server, then hit

12. Start Tomcat!
Part 3: Mobile App
1. Open
.
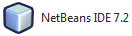
2. Click
to create a new project.

3. On the New Project dialog choose Java ME from the Categories then choose Mobile Application as the project type, then hit
.

4. Type EmployeeInfoMobile as project name, then hit
.

5. Enter the code below...
package hello;
import java.io.DataInputStream;
import javax.microedition.io.Connector;
import javax.microedition.io.HttpConnection;
import javax.microedition.lcdui.*;
import javax.microedition.midlet.MIDlet;
/**
* @author Jeremiah G. Gatong
*/
public class Midlet extends MIDlet implements CommandListener {
private Form f;
private Command find = new Command("Find", Command.OK, 1);
private TextField id;
private StringItem label;
public void main() {
f = new Form("Employee Information");
id = new TextField("Enter ID", "", 25, TextField.ANY);
label = new StringItem("", "");
f.append(id);
f.append(label);
f.addCommand(find);
f.setCommandListener(this);
}
public void startApp() {
main();
Display.getDisplay(this).setCurrent(f);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command c, Displayable d) {
if (c.equals(find)) {
StringBuffer sb;
try {
String url = "http://localhost:8080/EmployeeInfoWeb/Employee?id=" + id.getString();
HttpConnection cc = (HttpConnection) Connector.open(url);
cc.setRequestMethod(HttpConnection.GET);
cc.setRequestProperty("Content-Type", "text/plain");
DataInputStream in = (DataInputStream) cc.openDataInputStream();
int ch;
sb = new StringBuffer();
while ((ch = in.read()) != -1) {
sb.append((char) ch);
}
String str = sb.toString();
label.setText(str);
} catch (Exception e) {
System.err.println("Error :" + e);
}
}
}
}
Output
Subscribe to:
Posts (Atom)